ex1_scoreinput.html
va" style="width:100px"><br><br>
<b>HTML : </b>
<input type="text" id="txthtml" style="width:100px"><br><br>
<button type="button" id="btnresult" style="margin-left: 50px;">결과확인</button>
<div id="result" style="margin: 30px;">결과</div>
</div>
<script>
//자바점수를 입력후 포커스가 벗어날 때 발생하는 이벤트
document.getElementById("txtjava").onblur=function(){
//숫자가 아닐 경우 경고 후 포커스
if(isNaN(this.value))
{
alert("숫자로만 입력해주세요");
this.value="";//입력값 지우기
this.focus();//포커스 주기
return;//여기서 이벤트 함수를 종료한다
}
//0~100을벗어날 경우 경고표시 후 지우기
if(this.value<0 || this.value>100)
{
alert("0~100사이 값으로 입력해주세요");
this.value="";//입력값 지우기
this.focus();//포커스 주기
}
// if(!(this.value>=0 && this.value<=100)){
// alert("0~100사이 값으로 입력해주세요");
// this.value="";//입력값 지우기
// this.focus();//포커스 주기
// }
}
document.getElementById("txthtml").onblur=function(){
if(isNaN(this.value))
{
alert("숫자로만 입력해주세요");
this.value="";
this.focus();
return;
}
if(!(this.value>=0 && this.value<=100))
{
alert("0~100사이 값으로 입력해주세요");
this.value="";//입력값 지우기
this.focus();//포커스 주기
}
}
document.getElementById("btnresult").onclick=function(){
//이름
var irum=document.getElementById("txtname").value;
//자바점수
var java=Number(document.getElementById("txtjava").value);
//HTML 점수
var html=Number(document.getElementById("txthtml").value);
//총점
var total=java+html;
//평균
var avg=total/2;
//div의 요소값을 미리 얻는다
var r=document.getElementById("result");
//r에 결과값을 넣는다
// r.innerHTML="이름 : " +irum+ "<br>";
// r.innerHTML+="자바점수 : " +java+ "<br>";
// r.innerHTML+="HTML점수 : " +html+ "<br>";
// r.innerHTML+="총점 : " +total+ "<br>";
// r.innerHTML+="평균 : " +avg+ "<br>";
//또는 es6의 리터럴 문자열로 표시
var s=
`
이름 : ${irum}
자바점수 : ${java}
HTML점수 : ${html}
총점 : ${total}
평균 : ${avg}
`;
r.innerText=s;
}
</script>
</body>
</html>
(구현 화면)
ex2_imagesizechange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link
rel="stylesheet">
<style>
body * {
font-size: 1.2rem;
font-family: 'Single Day';
}
</style>
</head>
<body>
<div style="margin: 30px 30px;">
<h3>width 속성 변경하게 예제</h3>
<img src="../image/moviestar/12.jpg" id="photo1" width="200">
<br><br>
<button type="button" id="btn1">점점작게</button>
<button type="button" id="btn2">점점크게</button>
</div>
<script>
var photo1 = document.getElementById("photo1");
document.getElementById("btn1").onclick = function () {
var w = photo1.getAttribute("width");
console.log(w, typeof (w));//값과 속성
photo1.setAttribute("width", w - 10);
}
document.getElementById("btn2").onclick = function () {
var w = photo1.getAttribute("width");
console.log(w, typeof (w));//값과 속성
photo1.setAttribute("width", Number(w) + 10);
//eval로 해보기
//photo1.setAttribute("width",eval(w+"+"+10));
//photo1.setAttribute("width",eval(`${w}+10`));
}
</script>
<hr>
<div style="margin: 30px 30px;">
<h3>width 속성 변경하게 예제</h3>
<img src="../image/moviestar/3.jpg" id="photo2" style="width:200px">
<br><br>
<button type="button" id="btn3">점점작게</button>
<button type="button" id="btn4">점점크게</button>
</div>
<script>
var photo2 = document.getElementById("photo2");
document.getElementById("btn3").onclick = function () {
//현재 style의 width값을 얻어서 확인해보기
// var w=photo2.style.width;
// console.log(w,typeof(w));//200px string
var w = parseInt(photo2.style.width, 10);//10진수 숫자만 변환(10은 생략가능)
console.log(w, typeof (w));//200 number
photo2.style.width = (w - 10) + "px";
}
document.getElementById("btn4").onclick = function () {
var w = parseInt(photo2.style.width, 10);
console.log(w, typeof (w));
photo2.style.width = (w + 10) + "px";
}
</script>
</body>
</html>
(구현 화면)
ex3_fontchange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
#font{
margin: 50px 50px;
font-size: 24px;
font-family: 'Jua';
color:orangered;
}
</style>
<script>
//head 안에서 이벤트함수를 만들경우 반드시 onload 함수 안에서 구현한다
window.onload=function(){
var font=document.getElementById("font");
//글꼴 변경 이벤트
document.getElementById("selfont").onchange=function(){
font.style.fontFamily=this.value;
}
//글꼴 크기 변경 이벤트
document.getElementById("selsize").onchange=function(){
font.style.fontSize=this.value;
}
//글자색 변경 이벤트
document.getElementById("selcolor").onchange=function(){
font.style.color=this.value;
}
//체크 박스 이벤트
document.getElementById("chkfill").onclick=function(){
console.log("value:"+this.value); //value값 미지정시 on
console.log("check:"+this.checked); //true/false
if(this.checked)
font.style.backgroundColor="#add836";
else
font.style.backgroundColor="white";
}
//테두리선 이벤트
document.getElementById("chkborder").onclick=function(){
if(this.checked)
font.style.border=this.value;
else
font.style.border="";
}
}
</script>
</head>
<body>
<div style="margin: 10px 50px;">
<b>글꼴 변경 : </b>
<select id="selfont">
<option>Gamja Flower</option>
<option selected>Jua</option>
<option>Lobster</option>
<option>Single Day</option>
<option>Nanum Pen</option>
</select>
<br><br>
<b>글자크기 변경 : </b>
<select id="selsize">
<option>10px</option>
<option>14px</option>
<option>18px</option>
<option selected>24px</option>
<option>30px</option>
<option>40px</option>
<option>50px</option>
</select>
<br><br>
<b>글자색 선택 : </b>
<select id="selcolor">
<option>orangered</option>
<option>pink</option>
<option>#3cb371</option>
<option>#00bfff</option>
<option>rgb(122,100,200)</option>
<option>rgb(122,100,200,0.5)</option>
<option>#db7093</option>
</select>
<br><br>
(구현 화면)
ex4_기본문법연습.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h3>자바스크립트 연산자</h3>
<h4 style="background-color:orange">산술연산자 연습(+,-,*,/,%)</h4>
<script>
var su1=7;
var su2=4;
document.write("두수 더하기:"+(su1+su2)+"<br>");
document.write("두수 빼기:"+(su1-su2)+"<br>");
document.write("두수 곱하기:"+(su1*su2)+"<br>");
document.write("두수 나하기:"+(su1/su2)+"<br>");
document.write("두수 나머지:"+(su1%su2)+"<br>");
</script>
<h4 style="background-color: pink">증감연산자(++,--)</h4>
<script>
var a=3,b=3,m,n;
a++; //a값 1 증가, 단항일 경우 ++a, a++ 모두 같은 의미
++b;
document.write("a="+a+",b="+b+"<br>"); //4, 4
m=a++;//m=a; a++; 이 두줄과 같다. 즉 먼저 대입 후 a값이 증가된다.
document.write(`m=${m}, a=${a}<br>`);//4,5
n=++b; //b++, n=b b값이 먼저 증가된 후 n으로 대입된다
document.write(`n=${n}, b=${b}<br>`); //5,5
</script>
<h4 style="background-color: green;">대입연산자(=,+=,-=,*=,/=,%=)</h4>
<script>
var a=3;
a+=2; //a=a+2
document.write(a+"<br>");//5
a-=3; //a=a-3
document.write(a+"<br>");//2
a*=5;
document.write(a+"<br>");//10
a/=4;
document.write(a+"<br>");//2.5
a%=2;
document.write(a+"<br>");//0.5
</script>
</body>
</html>
ex9_loop.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body,body *{
font-size: 1.5rem;
font-family: 'Single Day';
}
.mycar{
width: 200px;
height: 130px;
}
.food{
width: 100px;
height: 100px;
border:3px inset hotpink;
margin-right: 10px;
}
</style>
</head>
<body>
<h2>구구단 5단 출력</h2>
<script>
for(i=1;i<=9;i++){
//document.write(`5 X ${i} = ${5*i}<br>`);
document.write("5 X "+i+" = "+5*i+"<br>");
}
</script>
<hr>
<h2>mycarimage 폴더에서 car1.png 부터 car10.png 까지 출력</h2>
<script>
for(i=1;i<=10;i++){
var s=`<img src="../image/mycarimage/car${i}.png" class="mycar">`;
document.write(s);
}
</script>
<h2>food 이미지에서 1.jpg~12.jpg 까지 출력(class="food") 로준후 style적용</h2>
<script>
for(i=1;i<=12;i++){
var s=`<img src="../image/food/${i}.jpg" class="food">`;
document.write(s);
}
</script>
</body>
</html>
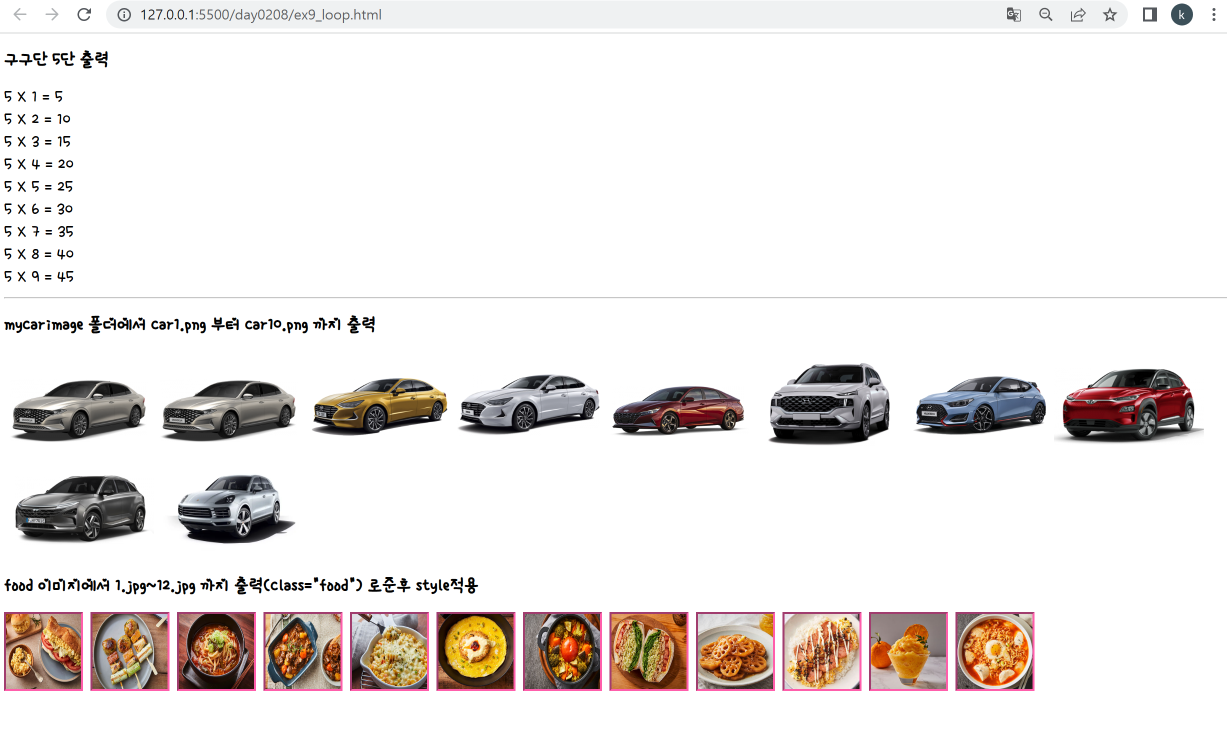
'학교 & 학원 이론 수업 > 네이버 클라우드 AIaaS 개발자 양성 과정' 카테고리의 다른 글
2023.02.10 금요일 - git , GitHub (0) | 2023.02.10 |
---|---|
2023.02.09 목요일 - 배열, table, for문, setTimeOut, setInterval (0) | 2023.02.09 |
2023.02.07 화요일 - CSS, HTML, JavaScript (0) | 2023.02.07 |
2023.02.06 월요일 - CSS, HTML, JavaScript (0) | 2023.02.06 |
2023.02.03 금요일 - HTML, CSS (0) | 2023.02.03 |