ex1_imagechange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body,body *{
font-size: 1.5rem;
font-family: 'Single Day';
}
#photo1{
width: 300px;
border:10px groove orange;
}
#starname{
margin-left: 100px;
font-size: 35px;
}
</style>
</head>
<body>
가나다
<h2>이미지의 속성을 변경하는 setAttribute,속성값을 얻는 getAttribute</h2>
<img src="../image/moviestar/19.jpg" id="photo1">
<br>
<b id="starname">수지</b>
<br>
<button type="button" id="btn1">현재값 얻기</button>
<button type="button" id="btn2">이미지랑 이름 변경하기</button>
<button type="button" id="btn3">원래대로</button>
<h2 id="msg">데이타 출력할곳...</h2>
<!--자바스크립트 이벤트들-->
<script>
var p1=document.getElementById("photo1");
var star=document.getElementById("starname");
var msg=document.getElementById("msg");
//버튼1 이벤트
document.getElementById("btn1").onclick=function(){
var s=p1.getAttribute("src");//사진1의 src속성값 얻기
var s2=star.innerText;//태그안의 text값을 얻는다
//문자열과 변수값을 조합해서 출력하는 방법 1
//msg.innerText="이미지:"+s+",이름:"+s2;
//문자열과 변수값을 조합해서 출력하는 방법 2
msg.innerText=`이미지:${s}, 이름:${s2}`;
}
// 버튼2 이벤트
document.getElementById("btn2").onclick=function(){
//p1의 속성인 src를 2번째 value 값으로 변경
p1.setAttribute("src","../image/moviestar/15.jpg");
//b 태그의 요소값을 msg변수로 얻어놨다
//그 변수의 text값을 변경하면 된다
star.innerText="설현";
}
// 버튼3 이벤트 - 원래대로
document.getElementById("btn3").onclick=function(){
p1.setAttribute("src","../image/moviestar/19.jpg");
star.innerText="수지";
}
</script>
</body>
</html>
(구동화면)
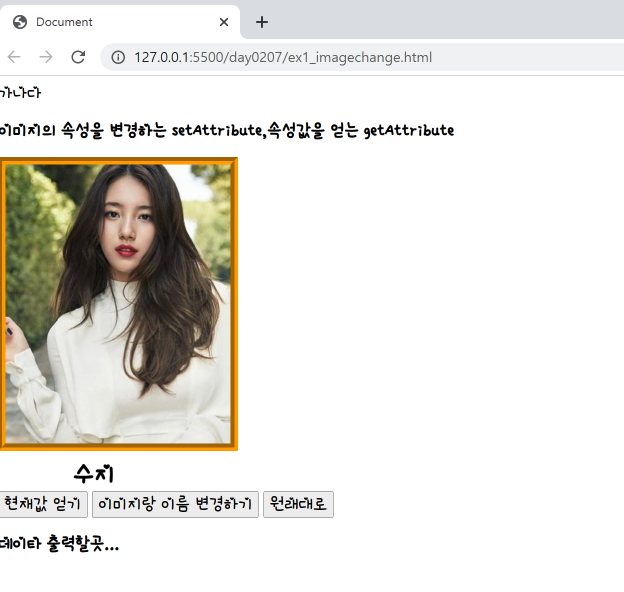
ex2_imagechange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body *{
font-size: 1.5rem;
font-family: 'Single Day';
}
#photo{
width: 300px;
height: 400px;
border: 10px inset gold;
}
figure{
text-align: center;
}
.buttons{
margin-left: 130px;
margin-top: 10px;
}
.buttons button{
width: 100px;
height: 100px;
background-color: plum;
color: blue;
border-radius: 30px;
cursor: pointer ;
}
.redline{
border-color: red;
}
.pinkline{
border-color: pink;
}
.radius30{
border-radius: 30px;
}
.radius60{
border-radius: 60px;
}
</style>
</head>
<body>
<figure>
<img src="../image/moviestar/13.jpg" id="photo" title="박신혜">
<figcaption>
<b id="name">박신혜</b>
</figcaption>
</figure>
<div class="buttons">
<button type="button" id="btn1">수지</button>
<button type="button" id="btn2">박보영</button>
<button type="button" id="btn3">유아인</button>
<button type="button" id="btn4">한예슬</button>
</div>
<script>
var p=document.getElementById("photo");
var irum=document.getElementById("name");
//버튼 1 이벤트 : 마우스 갖다대면 사진이랑 이름 바뀌게!
document.getElementById("btn1").onclick=function(){
//이미지 변경
p.setAttribute("src","../image/moviestar/19.jpg");
//이름 변경
irum.innerHTML="<b style='color:green'>수지</b>";
//출력할 이름을 prompt로 입력받아서 출력해보자
var myname=prompt("출력할 이름을 입력해주세요","수지");
//취소를 누를 경우 '이름을 입력해주세요'라고 출력하기
if(myname==null)
irum.innerText="이름을 입력해주세요";
else
irum.innerHTML=`<b style='color:green'>${myname}</b>`;
//사진의 title 속성 변경
p.setAttribute("title","수지입니다");
}
document.getElementById("btn2").onclick=function(){
//이미지 변경
p.setAttribute("src","../image/moviestar/12.jpg");
//이름 변경
irum.innerHTML="<b style='color:magenta'>박보영</b>";
//사진의 title 속성 변경
p.setAttribute("title","박보영입니다");
//사진의 class를 radius30으로 변경
p.setAttribute("class","radius30");
}
document.getElementById("btn3").onclick=function(){
//이미지 변경
p.setAttribute("src","../image/moviestar/20.jpg");
//이름 변경
irum.innerHTML="<b style='color:skyblue'>유아인</b>";
//사진의 title 속성 변경
p.setAttribute("title","유아인입니다");
//사진의 class를 radius60으로 변경
p.setAttribute("class","radius60");
}
document.getElementById("btn4").onclick=function(){
p.setAttribute("src","../image/moviestar/6.jpg");
irum.innerHTML="<b style='color:yellow'>한예슬</b>";
p.setAttribute("title","한예슬입니다");
//사진의 class 속성 없애기
p.setAttribute("class","");
}
(구동화면)
ex3_selectchange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body *{
font-size: 1.2rem;
font-family: 'Single Day';
}
</style>
</head>
<body>
<img id="animal" src="../image/animal/C1.png">
<br><br>
<select id="sel">
<option value="../image/animal/C1.png">귀염둥이고양이1</option>
<option value="../image/animal/C2.png">귀염둥이고양이2</option>
<option value="../image/animal/C3.png">귀염둥이고양이3</option>
<option value="../image/animal/C4.png">귀염둥이고양이4</option>
<option value="../image/animal/C5.png">귀염둥이고양이5</option>
<option value="../image/animal/C6.png">귀염둥이고양이6</option>
<option value="../image/animal/C7.png">귀염둥이고양이7</option>
<option value="../image/animal/C8.png">귀염둥이고양이8</option>
</select>
<script>
document.getElementById("sel").onchange=function(){
//this는 이벤트가 발생한 자기 자신을 나타내는 인스턴스 변수이다
//alert(this.value);
//2번째 방법은 event를 이용해서 얻는 방법
//e.target은 이벤트가 발생한 요소를 의미한다 (여기서는 this와 같은 의미)
//alert(e.target.value);
//이미지의 src를 select에서 얻은 값으로 변경해보자
document.getElementById("animal").setAttribute("src",this.value);
}
</script>
<br><br>
<b>선종류변경</b>
<select id="selborder">
<!--첫줄 고정 제목을 추가-->
<option selected disabled hidden>select border</option>
<option value="5px inset gray">테두리선 1</option>
<option value="10px inset orange">테두리선 2</option>
<option value="5px inset pink">테두리선 3</option>
<option value="1px inset green">테두리선 4</option>
<option value="5px inset gold">테두리선 5</option>
</select>
<script>
document.getElementById("selborder").onchange=function(){
document.getElementById("animal").style.border=this.value;
}
</script>
</body>
</html>
(구동화면)
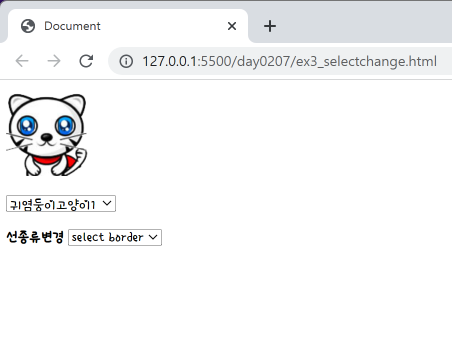
ex4_selectchange.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body *{
font-size: 1.2rem;
font-family: 'Single Day';
}
#box{
position: absolute;
left:200px;
top:100px;
width:200px;
height:200px;
border-width:10px;
border-style:solid;
border-color:cadetblue;
background-color: chartreuse;
}
.data{
position: absolute;
left:100px;
top:350px;
}
</style>
</head>
<body>
<div id="box"></div>
<div class="data">
<b>선스타일</b>
<select id="selstyle">
<option>solid</option>
<option>dotted</option>
<option>dashed</option>
<option>inset</option>
<option>groove</option>
<option>double</option>
</select>
<br><br>
<b>선색상</b>
<select id="selcolor">
<option selected disabled hidden>선색상 선택</option>
<option value="red">빨강색</option>
<option value="#ff69b4">핑크색</option>
<option value="#66cdaa">아쿠아색</option>
<option value="#9acd32">연두색</option>
<option value="#9400d3">보라색</option>
</select>
<br><br>
<b>채우기색상</b>
<select id="selbcolor">
<option selected disabled hidden>채우기색상 선택</option>
<option value="ffb6c1">연핑크</option>
<option value="green">그린색</option>
<option value="#8fbc8f">엔틱그린색</option>
<option value="afeeee">하늘색</option>
<option value="c71585">진핑크색</option>
</select>
</div>
<script>
var box=document.getElementById("box");
document.getElementById("selstyle").onchange=function(){
box.style.borderStyle=this.value;
}
document.getElementById("selcolor").onchange=function(e){
box.style.borderColor=e.target.value;
}
document.getElementById("selbcolor").onchange=function(){
box.style.backgroundColor=this.value;
}
</script>
</body>
</html>
(구동화면)
ex5_inputtype.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link href="https://fonts.googleapis.com/css2?family=Gamja+Flower&family=Jua&family=Lobster&family=Nanum+Pen+Script&family=Single+Day&display=swap" rel="stylesheet">
<style>
body *{
font-size: 1.2rem;
font-family: 'Single Day';
}
</style>
</head>
<body>
<div style="margin: 50px;">
숫자1
<input type="text" id="su1" style="width: 100px;">
숫자2
<input type="text" id="su2" style="width: 100px;">
<select id="sel">
<option selected disabled hidden>연산자 선택</option>
<option value="+">더하기</option>
<option value="-">빼기</option>
<option value="*">곱하기</option>
<option value="/">나누기</option>
</select>
<br><br>
<h1 id="result" style="text-align: center;"></h1>
<h1 id="result2" style="text-align: center;"></h1>
</div>
<script>
var su1=document.getElementById("su1");
var su2=document.getElementById("su2");
var se1=document.getElementById("sel");
///text안에 클릭시 발생하는 이벤트
su1.onfocus=function(){
this.style.backgroundColor="lightyellow";
}
//text에서 포커스가 벗어날 때 발생하는 이벤트
su1.onblur=function(){
this.style.backgroundColor="white";
//입력한 값이 숫자가 아닐 경우 경고 표시 후 값 지우고 포커스 주기
//console.log(isNan(this.value)); //숫자일경우 false. 문자면 true.
if(isNaN(this.value)) // ==true는 생략 가능
{
alert("숫자로만 입력해주세요");
this.value=""; //기존값 지우기
this.focus(); //포커스 주기
}
}
su2.onblur=function(){
//숫자가 아닐경우 조건문
if(isNaN(this.value))
{
alert("숫자로만 입력해주세요");
this.value="";
this.focus();
}
}
//select 이벤트
sel.onchange=function(){
//alert(this.value);
console.log(typeof(su1.value));
console.log(typeof(su2.value));
var r;
switch(this.value)
{
case '+':
//더하기일 경우에만 문자열 형태로 더한다
//제대로 계산되도록 하려면 숫자로 바꾼 후 계산해야 한다
//parseInt(정수로변환) 또는 parseFloat(실수로변환) 또는 Number(수치로변환)
//r=parseInt(su1.value)+parseInt(su2.value);
r=Number(su1.value)+Number(su2.value); //정수든 실수든 상관없이 숫자로 변환
break; //switch문을 빠져나간다, 생략시 다음 case가 실행
case '-':
r=su1.value-su2.value;
break;
case '*':
r=su1.value*su2.value;
break;
case '/':
r=su1.value/su2.value;
break;
}
document.getElementById("result").innerHTML=r;
//switch나 if로 계산없이 바로 계산결과 출력하기 -eval 이용
//eval("수식"):자동으로 계산해서 반환
var r2=su1.value+this.value+su2.value;
console.log(r2);
document.getElementById("result2").innerText=eval(r2); //수식r2를 계산해서 출력
}
</script>
</body>
</html>
(구동 화면)
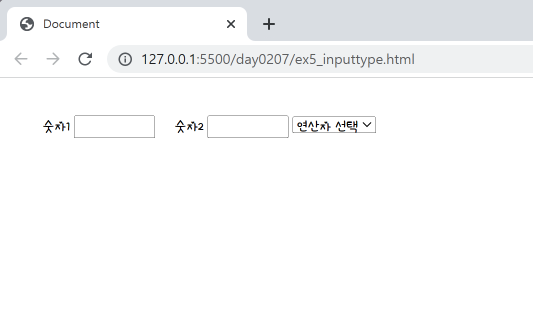
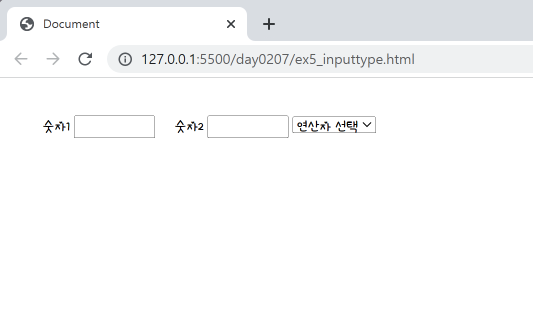
'학교 & 학원 이론 수업 > 네이버 클라우드 AIaaS 개발자 양성 과정' 카테고리의 다른 글
2023.02.09 목요일 - 배열, table, for문, setTimeOut, setInterval (0) | 2023.02.09 |
---|---|
2023.02.08 수요일 - getElementById, 연산자, 반복문 (0) | 2023.02.08 |
2023.02.06 월요일 - CSS, HTML, JavaScript (0) | 2023.02.06 |
2023.02.03 금요일 - HTML, CSS (0) | 2023.02.03 |
사전교육 3일차 (2023.02.02) (0) | 2023.02.02 |